Review
Write a static method called addThree that takes three int arguments and sums them.
Part One - What are arrays?
An array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. After creation, its length is fixed. You've seen an example of arrays already, in the main method of the "Hello World!" application.
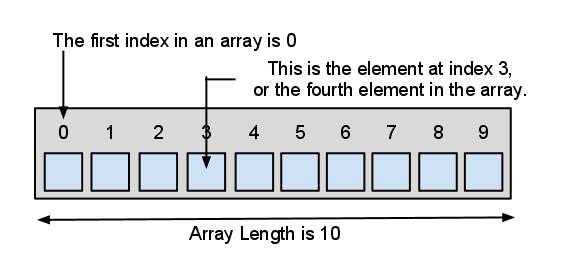
Each item in an array is called an element, and each element is accessed by its numerical index. As shown in the above illustration, numbering begins with 0. The 9th element, for example, would therefore be accessed at index 8
But why? Why does it have to start at 0?
Part Two - creating and accessing arrays
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | class ArrayDemo { public static void main(String[] args) { int [] anArray; // declares an array of integers anArray = new int [ 10 ]; // allocates memory for 10 integers anArray[ 0 ] = 100 ; // initialize first element anArray[ 1 ] = 200 ; // initialize second element anArray[ 2 ] = 300 ; // etc. anArray[ 3 ] = 400 ; anArray[ 4 ] = 500 ; anArray[ 5 ] = 600 ; anArray[ 6 ] = 700 ; anArray[ 7 ] = 800 ; anArray[ 8 ] = 900 ; anArray[ 9 ] = 1000 ; System.out.println( "Element at index 0: " + anArray[ 0 ]); System.out.println( "Element at index 1: " + anArray[ 1 ]); System.out.println( "Element at index 2: " + anArray[ 2 ]); System.out.println( "Element at index 3: " + anArray[ 3 ]); System.out.println( "Element at index 4: " + anArray[ 4 ]); System.out.println( "Element at index 5: " + anArray[ 5 ]); System.out.println( "Element at index 6: " + anArray[ 6 ]); System.out.println( "Element at index 7: " + anArray[ 7 ]); System.out.println( "Element at index 8: " + anArray[ 8 ]); System.out.println( "Element at index 9: " + anArray[ 9 ]); } } |
Lets break this thing down like we usually do.
int[] anArray; This is the declaration, here we are declaring an array of ints. we can also make an array of:
- String[]
- double[]
- boolean[]
- float[]
- or any other type or object
anArray = new int[10]; Here we allocate memory for the 10 elements that will go in the array. Notice that you put the size of the array inside the square braces.
Just so you know you can do both of those in one step like this int[] anArray = new int[10];
anArray[0] = 100; now, we are finally putting a value into the array
System.out.println("Element at index 0: " + anArray[0]); and here we access the element much like the way that we initialize it
Exercise 1
Create an array of Strings with length 3. Put your first middle and last names into the array. Then print them all.
Part Three - looping over arrays
Writing out a println statement for each element is a waste of time and not very flexible
If only we had a controll structure that you could use to do the same thing over and over agian...
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | class ArrayDemo { public static void main(String[] args) { int [] anArray; // declares an array of integers anArray = new int [ 10 ]; // allocates memory for 10 integers for ( int i = 0 ; i < anArray.length; i += 1 ) { anArray[i] = (i+ 1 ) * 100 ; } for ( int i = 0 ; i < anArray.length; i += 1 ) { System.out.println(anArray[i]); } } } |
Here you can see we used our counter as the index for our array. Thats why we've been using i, it stands for index
Exercise 2
Create an array of random numbers (doubles) between one and 10. Then loop through the array and count how many are less than 5
Part Four - array methods
Arrays are not objects so they have no methods. Fortunately for us Java provides a wrapper class with a bunch of static methods that we can use on arrays
Lets take a look at how a few of them work (these wont be on your lab test) :
import java.util.Arrays; public class ArrayMethods { public static void main(String[] args) { int[] arrayA = {2, 1, 3}; int[] arrayB = {1, 2, 3}; String[] strArray = {"greg","jon","alec"}; System.out.println( Arrays.equals( arrayA, arrayB ) ); //false Arrays.sort(arrayA); System.out.println(Arrays.toString(arrayA) ); // [1, 2, 3] System.out.println( Arrays.equals( arrayA, arrayB ) );//true Arrays.fill(arrayB, 0); System.out.println(Arrays.toString(arrayB) ); // [0, 0, 0] System.out.println(Arrays.binarySearch(arrayA, 2)); // 1 System.out.println(Arrays.binarySearch(arrayA, 42)); // -4 System.out.println(Arrays.binarySearch(strArray, "jon")); // 1 Arrays.sort(strArray); System.out.println(Arrays.toString(strArray)); // [alec, greg, jon] } }
Home Work
1. Loop over the following array and find the greatest number in it
[11, 4, 10, 2, 8, 11, 15, 7, 12, 5, 6, 1]
2. Using the same array, find the average
3. Using this list of names
["Leonard", "Sheldon", "Raj", "Howard", "Penny"]
Print the following output
Character number 1 is Leonard Character number 2 is Sheldon Character number 3 is Raj Character number 4 is Howard Character number 5 is Penny